You can ignore all the previous calculations like finding the distance of the camera to the close/far clipping plane using trig, scaling the vector by the ratio, calculating inverse matrix, and everything else that's be done before.
Now, everything's simple. 1 simple trig, and a couple of multiplications and divisions, and we're done. So basically, I just scratched what I've done in the past....2 weeks and went back to what I had originally done in the beginning of the project.
1) Find the dimensions of the far clipping plane
var farWidth = 2 * ((C3DL_FAR_CLIPPING_PLANE) * Math.tan(degreesToRadians(0.5 * C3DL_FIELD_OF_VIEW)));
var farHeight = farWidth * (tempDiv.height/tempDiv.width);
2) Find the ratio between the far clipping plane and the canvas window size
var wRatio = farWidth / tempDiv.width;
var hRatio = farHeight / tempDiv.width;
3) Finding the x,y coordinates of the mouse on the canvas window relative to the far clipping plane. And use the distance between the camera and the far clipping plane as the z value.
var farX = (currX / tempDiv.width) * farWidth;
var farY = (currY / tempDiv.height) * farHeight;
var farZ = C3DL_FAR_CLIPPING_PLANE - camPos[2];
To make the mouse vector be relative to where the camera is, we simply make the viewMatrix of the camera and multiply our mouse vector with it.
viewMatrix = makePoseMatrix(scn.getCamera().getLeft(), scn.getCamera().getUp(), scn.getCamera().getDir(), scn.getCamera().getPosition());
var tempMouseVec = makeVector(farX, farY, farZ);
tempMouseVec = multiplyMatrixByVector(viewMatrix, tempMouseVec);
So now, wherever I rotate or move my camera to, the mouse vector will always be shooting from what we see from where the camera is to where we see as the far clipping plane in the back of the canvas.
How did this process get simplify to such an extend, I must thank Cathy and our 1 hr discussion we had and all the diagrams we kept drawing on the white board. That discussion really helped me get a clear image of how the 3D world is shown in that 2D canvas window. What was causing the main problem for having the mouse vector always being skewed towards the center, is because we ended up doing multiple matrix transformations. For what we see on the canvas, the 3D space that we see (perspective) has already been skewed (transformed) so it's in a more rectangular form than a triangle, and then flatten before being put on the screen. I've drawn a simple diagram to show what's been done from a bird's eye view(please excuse the poor drawing).
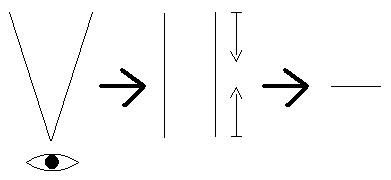
1) What we see in 3D space
2) Skewed (Perspective) and then Compressed
3) 2D (Canvas/Screen)
At least this is my understanding of it. And now that the mouse vector works properly, and FINAL (maybe minor improvements), I have to do the following:
1) Decide on an appropriate intersection test (have 2 right now)
2) Need to aligned mouse vector with testing object before testing intersection
3) Aligned object and mouse vector to axis to perform AABB test (Axis-Aligned Bounding Box)
I hope tomorrow's session with Andor will get these done. And afterward, I want to make the test do a more thorough intersection test rather than just the bounding box.
1 comment:
Excellent post...Thanks.
Post a Comment